Listen up, folks. If you're diving into the world of C++ programming, you've probably stumbled upon the term "double list STL." Now, before we dive deep, let me tell ya, this is no ordinary list. It's like the Swiss Army knife of data structures in C++ Standard Template Library (STL). Understanding it is like unlocking a secret weapon in your coding arsenal. So, buckle up because we're about to uncover what makes double list STL so darn powerful.
Imagine this: you're building a complex application that needs to handle dynamic data efficiently. That's where double list STL comes into play. It's like the backbone of your program, ensuring smooth data flow and manipulation. But don't just take my word for it. Stick around, and we'll break down everything you need to know about double list STL in a way that's easy to digest and packed with actionable insights.
Before we move forward, let’s get one thing straight. Double list STL isn't just for the coding wizards out there. It's for anyone who's serious about mastering C++ and taking their programming skills to the next level. Whether you're a beginner or a seasoned coder, there's something here for everyone. So, let's roll up our sleeves and dive right in.
Read also:Publix Super Market At Key Plaza Shopping Center Your Ultimate Shopping Destination
What Exactly is Double List STL?
First things first, let's demystify the concept of double list STL. Simply put, it's a container in C++ that allows you to store and manipulate a collection of elements. But here's the kicker - it's doubly linked, meaning each element points to both its predecessor and successor. This setup gives it an edge when it comes to inserting and removing elements at both ends, making it incredibly versatile.
Why Double List STL Stands Out
Here's the deal. While other data structures might lag when it comes to frequent insertions and deletions, double list STL thrives in such scenarios. It's like the hare in the tortoise vs. hare race when it comes to dynamic data handling. Let me break it down for you:
- Efficient Insertions: Adding elements at both the beginning and end is a piece of cake with double list STL.
- Quick Removals: Removing elements from either end is just as swift, making it perfect for queue and stack implementations.
- Bidirectional Access: You can traverse the list in both forward and backward directions, giving you unparalleled flexibility.
How Double List STL Works in C++
Alright, so now that we know what double list STL is, let's talk about how it operates under the hood. Think of it as a chain of nodes, where each node holds the data and pointers to its neighboring nodes. This structure enables seamless navigation and manipulation, making it a go-to choice for many programmers.
Key Features of Double List STL
Here's where things get interesting. Double list STL comes packed with features that make it a powerhouse in the world of data structures. Let's take a closer look:
- Dynamic Size: It can grow or shrink as needed, giving you the flexibility to handle varying amounts of data.
- Memory Efficiency: Unlike arrays, double list STL only allocates memory for the elements it contains, saving precious resources.
- Thread Safety: With proper synchronization, it can be safely used in multi-threaded environments, making it ideal for modern applications.
Applications of Double List STL
Now, you might be wondering where double list STL fits into the grand scheme of things. Well, its applications are as vast as the ocean. From managing playlists in music players to implementing undo-redo functionality in text editors, double list STL is everywhere. Let me give you a few examples:
- Browser History: It's used to keep track of visited pages, allowing users to navigate backward and forward effortlessly.
- Task Scheduling: In operating systems, it helps manage the order of tasks, ensuring smooth execution.
- Data Caching: It's employed in caching mechanisms to store and retrieve frequently accessed data efficiently.
Getting Started with Double List STL
Ready to roll up your sleeves and get your hands dirty? Great! Let's walk through the basics of using double list STL in C++. First off, you'll need to include the necessary headers and declare your list. Here's a quick rundown:
Read also:How Much Does Jesse Watters Make Unveiling The Salary Career And Net Worth
To get started, you'll need to include the header file. Then, declare your list using the appropriate data type. For instance, if you're dealing with integers, your list declaration would look something like this: list
Basic Operations with Double List STL
Once you've got your list up and running, it's time to perform some basic operations. Here's what you can do:
- Inserting Elements: Use the push_back() and push_front() functions to add elements at the end and beginning, respectively.
- Removing Elements: The pop_back() and pop_front() functions allow you to remove elements from either end.
- Traversing the List: Use iterators to move through the list, accessing each element as needed.
Advanced Techniques with Double List STL
Now that you've got the basics down pat, let's level up your skills with some advanced techniques. Double list STL offers a plethora of functions that can take your coding to the next level. Here's what you need to know:
Merging Lists
Sometimes, you'll need to combine two lists into one. Enter the merge() function. It takes two sorted lists and merges them into a single sorted list. It's like magic, but with code.
Sorting Lists
Sorting is a breeze with double list STL. The sort() function does the heavy lifting for you, arranging the elements in ascending order. Need it in descending order? Just provide a custom comparator.
Common Pitfalls and How to Avoid Them
Every coder knows that with great power comes great responsibility. While double list STL is a mighty tool, it's not without its pitfalls. Here are a few common mistakes to watch out for:
- Memory Leaks: Always ensure that you properly manage memory to avoid leaks.
- Null Pointers: Keep an eye out for null pointers when traversing the list.
- Thread Safety: If you're working in a multi-threaded environment, make sure to synchronize access to the list.
Best Practices for Using Double List STL
To get the most out of double list STL, it's essential to follow some best practices. Here's what the pros do:
- Profile Your Code: Use profiling tools to identify bottlenecks and optimize accordingly.
- Choose the Right Data Structure: Not every problem requires a double list. Sometimes, other structures might be a better fit.
- Document Your Code: Good documentation goes a long way in maintaining and understanding your codebase.
Real-World Examples of Double List STL
Talking theory is one thing, but seeing it in action is another. Let's take a look at some real-world examples where double list STL shines:
- Social Media Feeds: Platforms like Twitter use double list STL to manage user feeds, allowing for seamless scrolling and updates.
- File Systems: Operating systems employ it to manage file directories and their hierarchical structures.
- Networking Protocols: It's used in protocols like TCP to manage data packets efficiently.
Future Trends in Double List STL
As technology evolves, so does the role of double list STL. With the rise of parallel computing and cloud-based applications, its importance is only set to grow. Keep an eye on emerging trends and stay ahead of the curve.
Integration with Modern Frameworks
Modern frameworks are increasingly integrating double list STL into their cores, making it an indispensable part of the development process. It's like the peanut butter in a PB&J sandwich - essential and irreplaceable.
Conclusion
There you have it, folks. Double list STL is more than just a data structure; it's a powerhouse that can elevate your C++ programming skills to new heights. By understanding its mechanics and mastering its operations, you're well on your way to becoming a coding guru. So, what are you waiting for? Dive in and start exploring the endless possibilities that double list STL has to offer.
Now, here's the deal. If you found this article helpful, don't be shy to drop a comment or share it with your coding buddies. Knowledge is power, and together, we can conquer the world of programming. Happy coding, and see you in the next one!
Table of Contents
- What Exactly is Double List STL?
- Why Double List STL Stands Out
- How Double List STL Works in C++
- Applications of Double List STL
- Getting Started with Double List STL
- Advanced Techniques with Double List STL
- Common Pitfalls and How to Avoid Them
- Best Practices for Using Double List STL
- Real-World Examples of Double List STL
- Future Trends in Double List STL

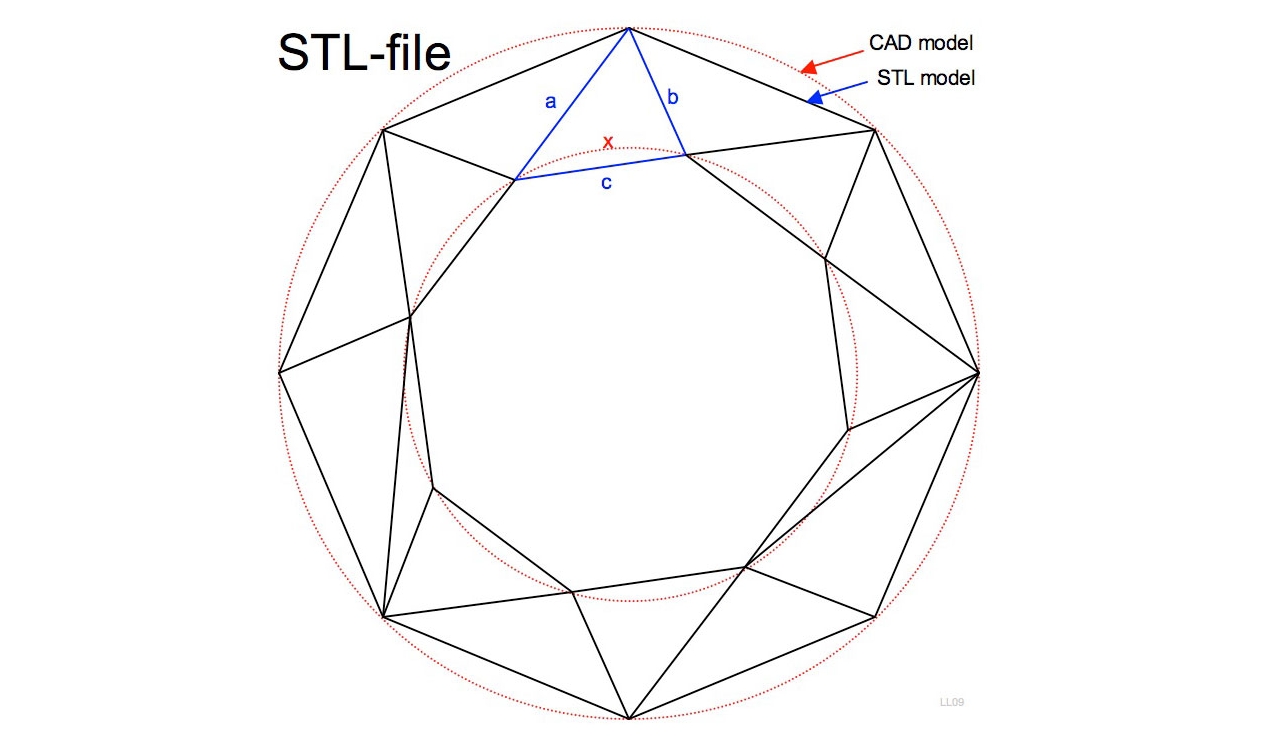
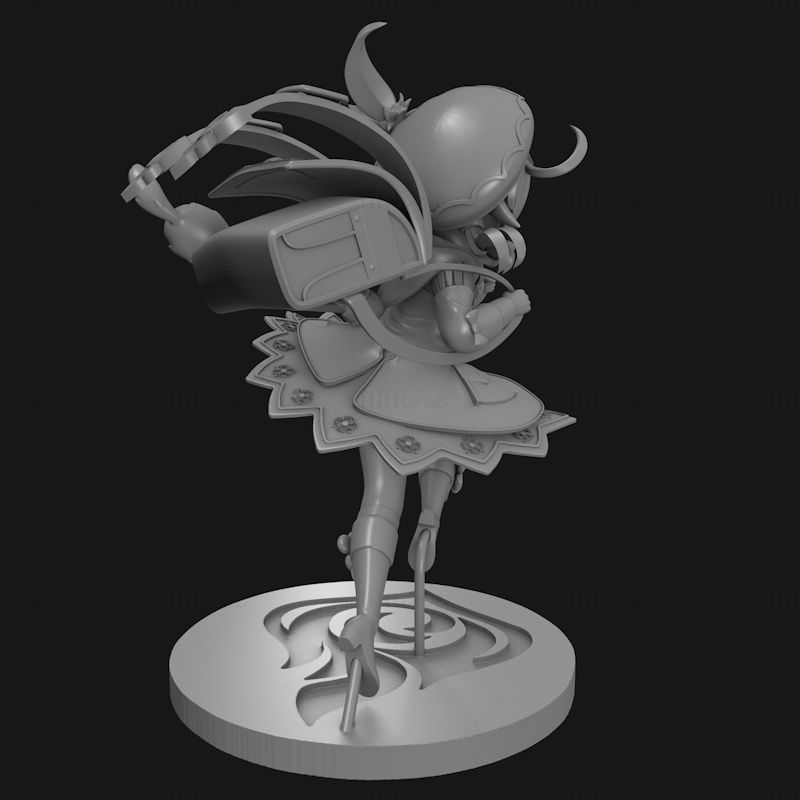